Introduction
Cross-Site Scripting (XSS) is a prevalent and dangerous security vulnerability found in many web applications. XSS attacks allow attackers to inject malicious scripts into web pages viewed by other users, potentially leading to data theft, session hijacking, and more. In this guide, we’ll explore how to fix XSS vulnerabilities and protect your website from these attacks.
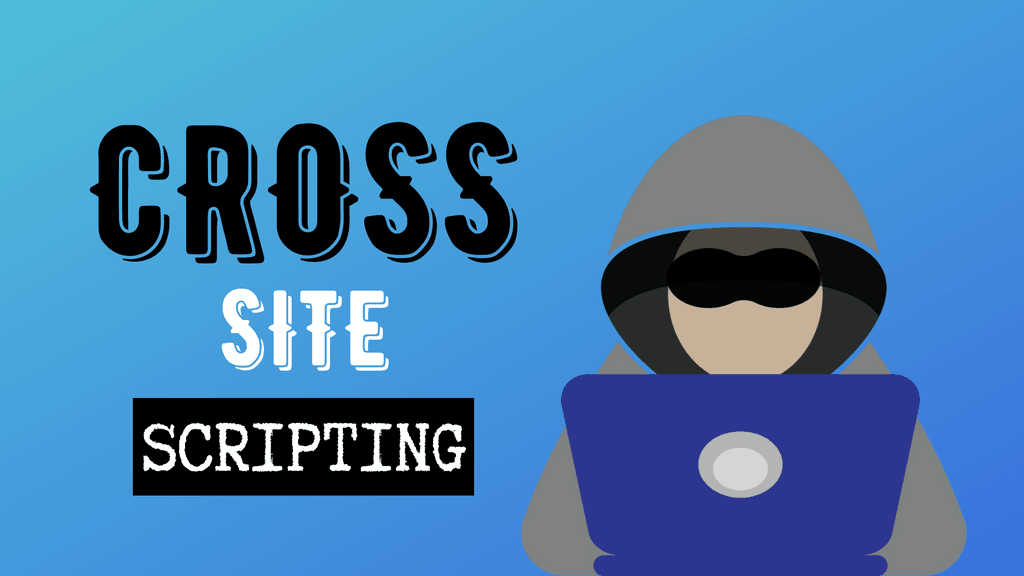
What is XSS?
XSS, or Cross-Site Scripting, occurs when an attacker injects malicious scripts into content from otherwise trusted websites. The browser executes these scripts because it assumes the content is safe, leading to various security issues. There are three main types of XSS attacks:
- Stored XSS: Malicious script is permanently stored on the target server, such as in a database.
- Reflected XSS: Malicious script is reflected off a web application to the user’s browser.
- DOM-based XSS: Malicious script is executed as a result of modifying the DOM environment in the victim’s browser.
Want to try it? This lab is vulnerable to XSS: Here
Why Fixing XSS is Crucial
Leaving XSS vulnerabilities unaddressed can lead to severe consequences, including:
- Data theft: Attackers can steal sensitive information from users.
- Session hijacking: Attackers can take over user sessions and perform actions on their behalf.
- Defacement: Attackers can alter the appearance of your website.
- Malware distribution: Attackers can use XSS to deliver malware to users.
How to Fix XSS
`Fixing XSS requires a multi-layered approach, involving both client-side and server-side techniques. Here are the key steps to mitigate XSS vulnerabilities:
1. Sanitize and Validate User Input
Sanitizing and validating user input is the first line of defense against XSS. Always treat user input as untrusted and sanitize it before processing.
$input = filter_input(INPUT_POST, 'user_input', FILTER_SANITIZE_STRING);
Use frameworks and libraries that provide built-in sanitization functions.
2. Escape Output
Escaping user input before displaying it in the browser prevents the execution of malicious scripts. Use functions like htmlspecialchars()
in PHP to escape HTML characters.
echo htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8');
In JavaScript, use functions like textContent
to prevent script execution.
element.textContent = user_input;
3. Implement Content Security Policy (CSP)
A Content Security Policy (CSP) helps prevent XSS by specifying which sources are allowed to load content on your site.
header("Content-Security-Policy: default-src 'self'; script-src 'self' https://trusted.cdn.com;");
4. Use HTTPOnly and Secure Cookies
Set the HttpOnly
and Secure
flags on cookies to prevent access to cookie data via JavaScript and ensure cookies are only sent over HTTPS.
setcookie('session', $value, [
'httponly' => true,
'secure' => true,
'samesite' => 'Strict'
]);
5. Regularly Update Software
Keep your web application, libraries, and frameworks updated to patch known vulnerabilities. Regular updates reduce the risk of XSS and other attacks.
6. Disable Inline JavaScript
Avoid using inline JavaScript and eval()
functions, as they are more susceptible to XSS attacks. Use external scripts and Content Security Policies to control script execution.
7. Educate Your Team
Ensure that your development team is aware of XSS risks and trained in secure coding practices. Regular security training can help prevent common vulnerabilities.
Watch This Video for a Detailed Walkthrough to fix xss
For a visual guide, watch this YouTube video: How to fix XSS.
Conclusion
Mitigating XSS vulnerabilities is essential for maintaining the security and integrity of your web applications. By following the steps outlined in this guide, you can significantly reduce the risk of XSS attacks and protect your users from malicious scripts. Regularly review and update your security practices to stay ahead of emerging threats.
Key Takeaways to fix xss
- Sanitize and validate all user inputs.
- Escape outputs to prevent script execution.
- Implement a robust Content Security Policy.
- Use HTTPOnly and Secure cookies.
- Keep software and frameworks updated.
- Avoid inline JavaScript and
eval()
. - Educate your team on secure coding practices.
By adopting these best practices, you can effectively fix XSS vulnerabilities and ensure a safer web experience for your users.